Learning Goals
At the end of this Tutorial, you will be able to:
- Create a function declaration.
- Create a function expression.
- Recognise that function declarations are hoisted but function expressions are not.
- Recognise that function expressions may be unnamed or anonymous.
- Use arrow functions that contain one or multiple statements.
About functions
Functions are the building blocks of a programming language. You can think of a function as a ‘box’ or ‘sub-program’ that stores some code inside it.
In summary:
- A function is a block of code that performs a particular task.
- Whenever the program needs that task to be performed, it invokes (‘calls’ or ‘fires’) the function and runs the code inside it.
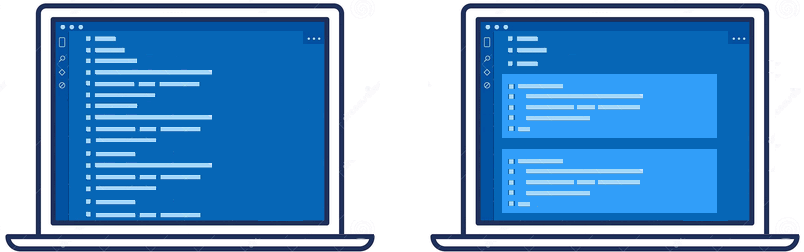
Dividing your program into functions brings one huge advantage: You need to write the code inside functions only once – but the program can invoke and run the functions as many times as is needed.
In short, functions are reusable and recyclable ♻️ code blocks.
Function names
In a JavaScript program, you can call a function by typing its name, followed by a pair of parenthesis () and a semi-colon ; For example:
displayGreeting();
There is no space between the function name and the opening parenthesis.
Here are some important points about naming functions in JavaScript:
- Names must be unique: Not every function need to have a name. (Functions can be anonymous.) But when a function has a name, that name must be unique. No two functions in the same scope may have the same name.
- No spaces or hyphens: Function names may not contain spaces or the hyphen character (-). The underline character (_) may be used and often is.
- Mixed character case: Developers typically combine upper and lowercase letters in a function name to make the name easier to read. For example, setFontSize() and calculateTax(). This convention is known as camelCase.
- Case sensitivity: Function names are case-sensitive. UpdateTotal() and updateTOTAL() are two different function names.
It's always a good idea to start a function name with an imperative verb (an action word such as ‘add’, ‘sum’, ‘calculate’, ‘update’, ‘display’ or ‘push’) that describes what the function does.
Functions and code blocks
In JavaScript, a code block is some code inside a pair of braces {}, also known as curly brackets. The code of a function is called the function body and is always written inside a pair of braces.
Typically, the code inside the code block is indented from the left by two spaces to make the function easier to read.
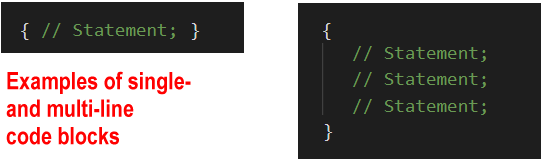
JavaScript functions may be created in different ways. Two of the most common are function declarations and function expressions. See below.
Function declarations
One way to create a function is to declare it with the relevant JavaScript keyword, much in the same way you would declare a variable.
- You declare variables with the var, const or let keywords.
- You declare a function with the function keyword.
See the sample function declaration below:
// Function declaration function displayGreeting() { console.log("Hello, world!"); }
Here is the syntax of a function declaration:
- Begins with the function keyword
- Then, after a space, comes the function name
- Then, without a space, is a pair of parentheses (). This may include one or more parameters separated by commas.
- Finally, inside a code block {}, you type the function's code.
You would call this function as shown below.
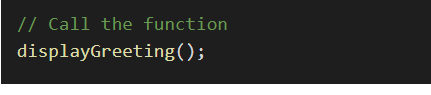
Function declarations and coding styles
Developers write function declarations in slightly different ways.
- The space after the pair of parenthesis () and before the opening brace { of the code block is optional.
- And some developers begin the code block {} on a new line. All three examples below will run correctly.
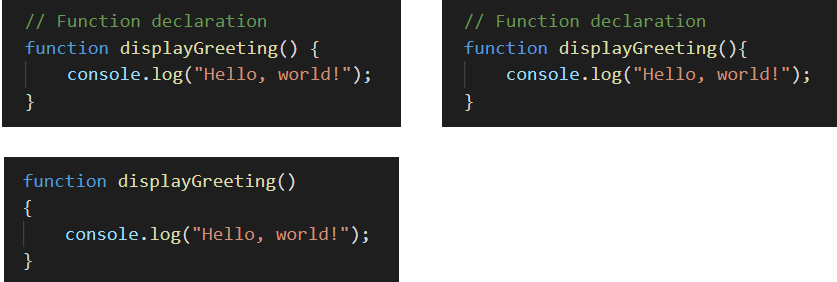
Function declarations are hoisted
A function declaration creates a function variable (with the same name as the function name) in the current scope.
Function variables are hoisted. As a result, you can call them before or after they are declared. Both of the code samples below will run without error.
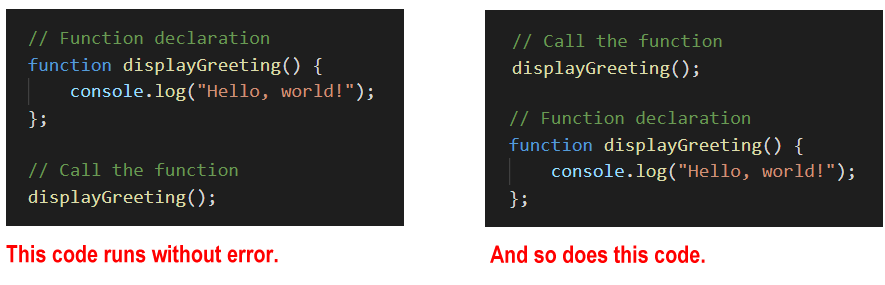
Function expressions
A second way to create a function is with a function expression. See the example below:
// Function expression const greeting = (function displayGreeting() { console.log("Hello, world!"); });
A function expression is stored inside a regular JavaScript variable.
The syntax of a function expression as follows:
- Begin by writing a function declaration as shown below
- Enclose the function declaration inside a pair of parenthesis () .
- Add a semi-colon statement terminator ; at the end.
A function expression ends with a semi-colon because it is a part of an executable statement.
- Begin the code with an equals = sign to make it the RHS of an assignment statement.
- On the LHS, write the variable keyword and the name of the variable that will store the function.
Developers typically use the const variable type to store a function expression. This is to ensure the function is not accidentally changed or overwritten elsewhere in the program.
Note the following:
- A function declaration begins with the function keyword.
- A function expression includes the function keyword, but begins with a variable declaration.
Strictly speaking, a function expression is not stored inside a JavaScript variable. Only the the value that the function expression evaluates to is stored in the variable.
Function expressions are not hoisted
You call a function expression in a similar way to how you call a function declaration. The difference is that you type the variable name rather than the function name. As with calling a function declaration, you follow this, without a space, with a pair of parenthesis (). See the example below.
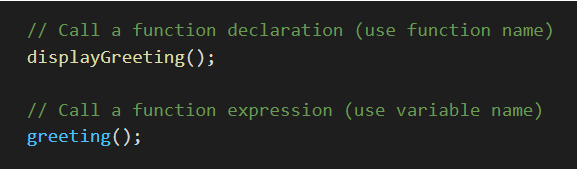
Here are two important differences between function declarations and function expressions:
- Unlike a function declaration, a function expression does not create a function variable (which is hoisted).
- Instead, the function is stored inside a regular variable (which is not hoisted).
As a result, you can call a function expression only after you have created it – and never before.
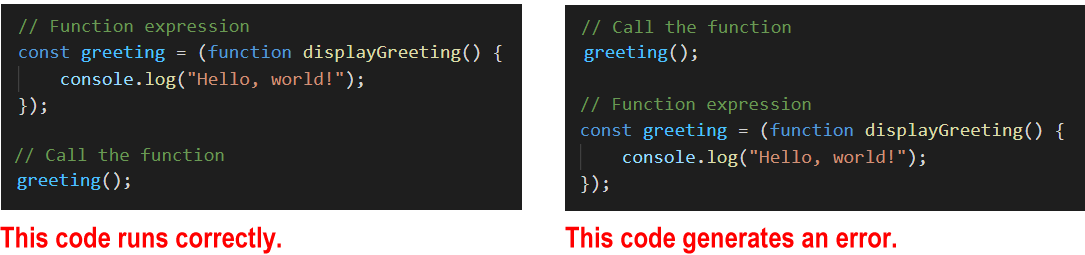
In other words, you must treat a variable that stores a function expression in the same way as any other variable – first declare it, and only then use it.
Anonymous function expressions
Consider the sample function expression below.
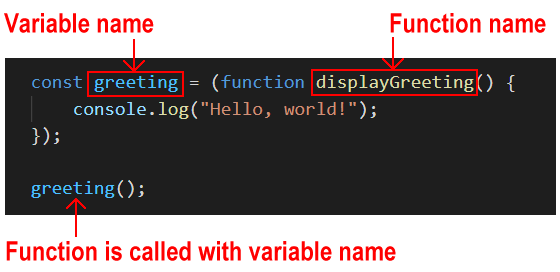
You can see that the function contains two names:
- A variable name: In this example, greeting.
- A function name: In this example, displayGreeting.
But only one name is needed to call the function elsewhere in the program – the variable name.
So: do we need the function name at all?
Answer: 'No'.
You can convert a named function expression to an unnamed or anonymous function expression as follows:
- Remove the function name. But leave the parenthesis () after the keyword function.
- Remove the outer parenthesis () that encloses the RHS of the assignment statement.
That's it. You have now created an anonymous function.
Arrow function expressions
A so-called arrow function is a short-hand or quick way of typing an anonymous function expression.
Because arrow functions are always anonymous, you must assign them to a variable if you ant to call them from elsewhere in the program.
You can convert a regular anonymous function to an arrow function as follows:
- Remove the function keyword. But leave the parenthesis ().
- After the () and before the opening code block {, type an equals sign and a right arrow character =>
That's it. You have now created an arrow function.
For a function expression that contains only one statement, you can make the syntax of the arrow function even shorter. Here are the steps:
- Place all the function code on one line.
- Remove the code block braces {} and the final statement terminator ;.
This is possible only for single-statement functions. Arrow functions with multiple statements still need to include a code block {} that ends with a ; terminator.
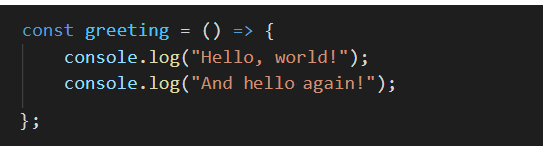
Notice that, with arrow functions, the JavaScript syntax for declaring and initializing variables, and for creating function expressions, is very similar.
In both cases, the LHS of the assignment statement contains a variable type such as const and a variable name.
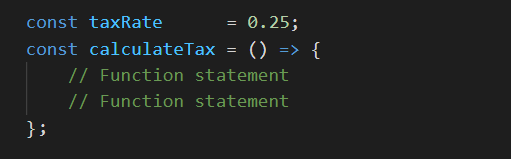
One difference is that, for arrow functions, the RHS of the assignment statement begins with the () => characters.
Another difference is that, unless the arrow function contains only one statement, the RHS will also contain code block braces {}.