Project Description
An increment and decrement counter that changes the colour of the number displayed on the web page.
You can view a completed version of the project here.
Your project folder
In your portfolio/js folder is a folder named counter that contains all the files you need for this JavaScript project.
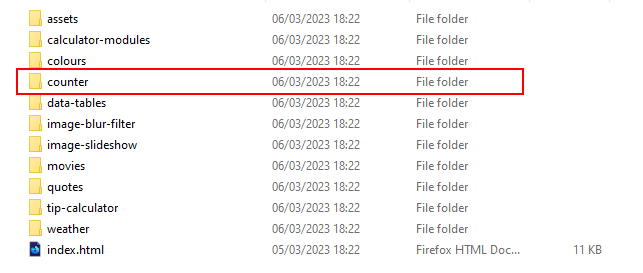
In VS Code, open the project’s index.html web page and ensure the hyperlinks and personal details have been correctly updated.
Adding the JavaScript code
Follow the steps below to add the JavaScript code to the index.html file.
- Scroll down the web page until you see an empty pair of opening <script> and closing </script> tags.
- Click inside the empty pair of tags and paste the JavaScript code below.
// set inital value to zero let count = 0; // select value and buttons const value = document.querySelector("#value"); const btns = document.querySelectorAll(".btn"); btns.forEach(function (btn) { btn.addEventListener("click", function (e) { const styles = e.currentTarget.classList; if (styles.contains("decrease")) { count--; } else if (styles.contains("increase")) { count++; } else { count = 0; } if (count > 0) { value.style.color = "green"; } if (count < 0) { value.style.color = "red"; } if (count === 0) { value.style.color = "#222"; } value.textContent = count; }); });
- Save your index.html file.
- Display the file in a web browser and verify it works correctly.
Uploading your project to GitHub
Upload the counter folder as a sub-folder of the portfolio/js folder on your GitHub account.
Learning more with ChatGPT
Follow the steps below to learn more about this JavaScript code from ChatGPT:
- In ChatGPT, enter the following prompt:
Explain in simple terms the following JavaScript code
- Press the Shift + Enter keys twice.
- Paste the JavaScript code and click the arrow icon.
Here are some more chatGPT prompts you may find helpful.
Use a step-by-step list to explain the code
Create documentation for this code
Create a basic tutorial for a JavaScript student based on this code
What does this code show about how events works JavaScript
What does this code show about how event listeners work JavaScript
What does this code show about how the forEach loop works JavaScript
What does this code show about how arrays in JavaScript
What does this code show about selecting elements in a web page
Finally, ask ChatGPT to create its own version of this JavaScript project.
In JavaScript create an increment and decrement counter that changes the colour of the number displayed on a web page. Please include some helpful documentation.
Compare this code with the code you have entered above.