Learning Goals
At the end of this Tutorial, you will be able to:
- Use the array.map() method to apply a function to every item in a copy of an array.
- Use single and multi-line arrow functions, and external functions, within the array.map() method.
In your javascript/exercises folder, create a new sub-folder named array-map.
Save the exercise file below to this new javascript/exercises/arrays-intro sub-folder.
The array.map()
method
Use the array.map() method to:
- Make a copy of an array, and
- Run a function on each item in the new, copied array.
The original array is unaffected.
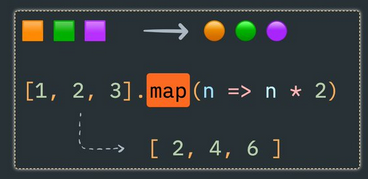
The general syntax is a follows:
const newArray = oldArray.map(arg)
The arg argument passed to the .map() method could be:
- A one-line arrow function. No return statment is needed.
- A multi-line arrow function with code wrapped inside a {}. You need to include a return statement if you want the output to be used elsewhere in the code.
- An external function declared elsewhere in the code.
Mapping arrays with one line-arrow functions
See the following:
myArray = ['apple', 'banana', 'orange'];
// Capitalise each item in the copied array
const myList = myArray.map(item => item.toUpperCase());
console.log(myList);
// Outputs ['APPLE', 'BANANA', 'ORANGE']
In the first example below, each item in the copied array is doubled by the arrow function inside the loop. In the second example, each item in the copied array has the number three added to it.
const numbers = [1, 2, 3, 4, 5];
// Multiply each item in the copied array by 2
const doubled = numbers.map(number => number * 2);
console.log(doubled);
// Outputs [2, 4, 6, 8, 10]
// Add 3 to each item in the copied array
const plusThree = numbers.map(number => number + 3);
console.log(plusThree);
// Outputs [4, 5, 6, 7, 8]
Mapping arrays with multi-line arrow functions
Use the multi-line format when the transformation logic inside the .map() loop is a bit more complex. See the following:
const celsiusTemps = [0, 25, 30, 100];
const fahrenheitTemps = celsiusTemps.map(temp => {
// Assign each item of new array to a variable named temp
const fahrenheit = (temp * 9/5) + 32;
return `${temp}°C is ${fahrenheit}°F`;
});
console.log(fahrenheitTemps);
// Outputs: ["0°C is 32°F", "25°C is 77°F", "30°C is 86°F", "100°C is 212°F"]
Note that you're not re-declaring the fahrenheit variable in the same scope multiple times. On each iteration through celsiusTemps array, a new scope is created by the {}, allowing for a new fahrenheit variable to be declared without any conflicts with the previous one. In short, you're declaring the variable in different, unique scopes for each iteration of the loop.
Mapping arrays with external functions
When the transformation logic is complex or needs to be reused elsewhere in the code, it makes sense to place it in a separate function outside the .map() loop. This pattern can make your code more modular and readable.
See the example below.
// Original array of radius values
const radii = [5, 10, 15];
// Function to calculate the area of a circle
function circleArea(radius) {
return Math.PI * radius * radius;
}
// Apply the area calculation function to each item in the new array named radii
const areas = radii.map(circleArea);
console.log(`Circle 1 radius: ${radii[0]} Circle area: ${areas[0].toFixed(4)}`);
console.log(`Circle 2 radius: ${radii[1]} Circle area: ${areas[1].toFixed(4)}`);
console.log(`Circle 3 radius: ${radii[2]} Circle area: ${areas[2].toFixed(4)}`);
// Outputs:
// Circle 1 radius: 5 Circle area: 78.5398
// Circle 2 radius: 10 Circle area: 314.1593
// Circle 3 radius: 15 Circle area: 706.8583
In this example, for each item in the array:
- The .map() method calls the circleArea() function, and
- Passes the array item as the input parameter to that function.
The function is not immediately invoked by .map() but is passed by reference to it as an argument and runs outside the .map() loop. The function's output is then returned to the loop.
You could rewrite this as a one-line arrow function as follows:
const areas = radii.map(radii => { return Math.PI * radii * radii });
Or even more concisely as follows:
const areas2 = radii.map(radii => Math.PI * radii * radii);
Mapping arrays of objects
You can use the .map() method with arrays of objects. See the two examples below.
const users = [
{ firstName: "Susan", lastName: "Steward", age: 14, hobby: "Singing" },
{ firstName: "Daniel", lastName: "Longbottom", age: 16, hobby: "Football" },
{ firstName: "Jacob", lastName: "Black", age: 15, hobby: "Singing" }
];
// In the copied array the firstName and lastName item properties are output together
const fullNames = users.map(user => console.log(`${user.firstName} ${user.lastName}`));
// Outputs:
// Susan Steward
// Daniel Longbottom
// Jacob Black
const products = [
{ id: 1, name: 'Laptop', price: 1000 },
{ id: 2, name: 'Mouse', price: 50 },
{ id: 3, name: 'Keyboard', price: 70 }
];
// Apply 10% discount to each item in the copied array
const specialOffers = products.map(product => {
console.log(`${product.name} (id: ${product.id}) Sale price! €${product.price * 0.9}`);
});
// Outputs:
// Laptop (id: 1) Sale price! €900
// Mouse (id: 2) Sale price! €45
// Keyboard (id: 3) Sale price! €63