Learning Goals
At the end of this Tutorial, you will be able to:
- Declare string variables in JavaScript and use typeof to test that a variable contains a string.
- Concatenate strings with the + operator and split them with the slice() method.
- Use index position and the .length property to work with string characters.
- Remove whitespace with trim() and change character case with toUpperCase() and toLowerCase().
- Use various methods to query a string by index position and character(s).
- Search and replace string characters.
There is no exercise file for this Tutorial.
Introducing strings
A string is a sequence of one or more characters – letters, numbers, or symbols. Each character in a string can be accessed by its position-based index number, beginning with zero. All strings have methods and properties available to them.
JavaScript offers two types of strings:
- String primitives
- String objects
This Lesson focuses only on the more commonly-used string primitives.
Assigning strings to variables
To assign a string to a variable, wrap the value in single or double quotes.
const myStr1 = "Hello"; // JavaScript treats myStr1 as a string const myStr2 = 'World'; // JavaScript treats myStr2 as a string const myStr3 = " "; // JavaScript treats myStr3 as a string
Note that a whitespace character is treated as a valid string. The myStr3 variable is not empty.
With the let keyword, an empty string can be declared (created) in one statement and then initialised (first value assigned to it) in another.
let myStr4 = ""; // Empty string
myStr4 = "Some text here"; // String now has a value
You cannot declare an empty string with const. An error will result.

A string declared with let (but not const) can be reassigned.
let myStr5 = "Things are looking good.";
myStr5 = "Things are looking even better."; // New value assigned
Attempting to reassign a variable created with const throws an error as shown below.

The typeof
operator
If you are unsure about a variable’s data type, you can use the typeof operator on the variable as shown below.
// all statements returns string
console.log(`Data type of myStr1: ${typeof myStr1}`);
console.log(`Data type of myStr2: ${typeof myStr2}`);
console.log(`Data type of myStr3: ${typeof myStr3}`);
console.log(`Data type of myStr4: ${typeof myStr4}`);
console.log(`Data type of myStr5: ${typeof myStr5}`);
Strings and index position
JavaScript assigns an index number to each character in a string, starting with 0. This is called zero-based indexing. See below.
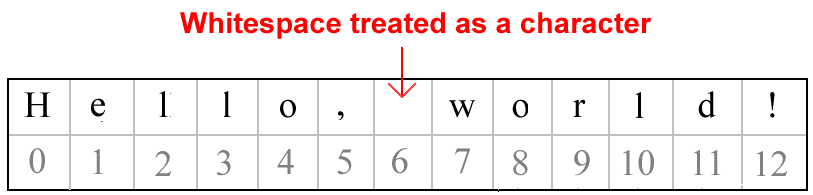
See the sample code below.
let strHelloWorld = "Hello, World!"; console.log(`Position [0]: ${strHelloWorld[0]};`) // returns H console.log(`Position [5]: ${strHelloWorld[5]};`) // returns , console.log(`Position [6]: ${strHelloWorld[6]};`) // returns whitespace console.log(`Position [7]: ${strHelloWorld[7]};`) // returns W console.log(`Position [12]: ${strHelloWorld[12]};`) // returns !
The first character in the string is H corresponds to index position 0. The last character is !, which corresponds to index position 12. The whitespace character also have an index, at 6.
Being able to access every character in a string with the square bracket [] notation makes it easy to access and manipulate strings.
The length of a string
Every string has a length property that reveals the number of characters in the string. The syntax is as follows.
string_name.length
The following example will output a string length of 13 to the console.
console.log(Number of chars in strHelloWorld: "+strHelloWorld.length);
// returns 13
Remember: the length of a string is always one number greater than the string’s highest index number.
Trimming whitespace from a string
Users of online forms can enter unnecessary whitespace characters at the start or end of an input field. To remove these spaces, use the following JavaScript method. These spaces are called padding.
.trim() |
Returns a new string without any whitespace characters at the start and end of the string. |
This method will return a new string. This means the original string will be unchanged. So you will probably want to reassign the string variable to the new, trimmed value as follows.
See the sample code.
let strUserFirstName = " Marie ";
strUserFirstName = strUserFirstName.trim();
console.log(`Trimmed string variable: ${strUserFirstName};`)
// returns Marie
In addition to whitespaces, trim() also removes tab, no-break space and line terminator characters.
Note that trim() only works for characters at the start and end of a string. Any spaces between words are unaffected.
Changing the case of string characters
You can use the following methods to change the case of a string.
Because each method actually creates a new string, you will typically want to reassign the converted values back to the original strings.
.toUpperCase() |
Returns a new string of all upper-case characters. |
.toLowerCase() |
Returns a new string of all lower-case characters. |
See the sample code below.
let strMakeUpper = "U2\'s First Album"; let strMakeLower = "U2\'s Second Album"; strMakeUpper = strMakeUpper.toUpperCase(); console.log(`All Uppercase String One: ${strMakeUpper}`); // returns U2's SECOND ALBUM strMakeLower = strMakeLower.toLowerCase(); console.log(`All Lowercase String Two: ${strMakeLower}`); // returns u2's second album
Joining strings together
You can use the plus + operator to concatenate (join) strings together into one string. Often you will want to include a whitespace character before or after the + when joining strings.
See the examples below.
console.log(`"John"+"Lennon": ${"John"+"Lennon"}`); // returns JohnLennon console.log(`"John "+"Lennon": ${"John "+"Lennon"}`); // returns John Lennon console.log(`"John" +" Lennon": ${"John" +" Lennon"}`); // returns John Lennon
JavaScript also offers a concat(a,b) method. But this is best avoided because it will throw an error if arguments passed are not string variables. The + operator will coerce any non-string values to strings.
Slicing strings apart
The opposite of concatenating strings is to splice a string. That is, to break a single string into two string variables. You can use the following method.
.slice(start,end) |
Returns a new string extracted at the specified start position from the original string. If the optional end argument is omitted, the new string will include every character of the original string up to the end. |
See the examples below.
let strForSplicing = "A Long Day\'s Journey Into Night"; console.log(`Splice at position [6] to end: ${strForSplicing.slice(6)}`); // returns Day's Journey Into Night console.log(`Splice from position [7] to [10]: ${strForSplicing.slice(7,10)}`); // returns Day
The original string is unchanged.
Querying a string by index position(s)
Do you need to know what character(s) occur at particular index positions(s) in a string? JavaScript provides three methods to answer that question.
All three methods below are case-sensitive and create a new string containing the returned result. The tested string is unchanged.
.charAt(index) |
Returns the character at the specified index position. |
Here are two simple examples.
const strMovieTitle = "The Man Who Shot Liberty Valence"; console.log(`Character at position [0]: ${strMovieTitle.charAt(0)}`); // returns T console.log(`Character at position [17]: ${strMovieTitle.charAt(17)}`); // returns l
substring(start, end) |
Returns the string character(s) between start and end index positions, but not including the end position. Omit the end argument to include all characters from the start to the end of the string. |
See an examples below.
console.log(`Characters from position [0] to before position [3]: ${strMovieTitle.substring(0,3)}`); // returns The console.log(`Characters from position [4] to string end: ${strMovieTitle.substring(4)}`); // Man Who Shot Liberty Valence
substr(start, length) |
Returns the string character(s) from the specified start position. The optional length parameter sets an ending index position. |
See an example below.
console.log(`3 Characters, beginning at position [0]: ${strMovieTitle.substr(0,3)}`); // returns The console.log(`8 Characters, beginning at position [4]: ${strMovieTitle.substr(4,8)}`); // returns Man Who
If you supply these methods with arguments that are out of range, JavaScript returns an empty string "".
Querying a string for character(s)
Often you will want to know if a string contains particular character(s).
In the two simplest cases, where the search-for characters(s) are at the start or the end of the string, you can use one of these methods.
.startsWith(searchString) |
Returns true if a string begins with specified character(s). |
.endsWith(searchString) |
Returns true if a string ends with specified character(s). |
See the code samples below.
console.log(`Does Movie Title begin with "Th": ${strMovieTitle.startsWith("Th")}`); // returns true console.log(`Does Movie Title end with "VALENCE": ${strMovieTitle.endsWith("VALENCE")}`); // returns false. Search is case-sensitive
More generally, you can test if character(s) are located anywhere in a string with this method.
.includes(searchString, position) |
Tests if the specified character(s) occur anywhere in a string. The optional position parameter begins the search at a particular index value. Its default value is 0. |
Here are some examples.
console.log(`Does Movie Title include "Shot" anywhere? ${strMovieTitle.includes("Shot")}`); // returns true console.log(`Does Movie Title include "The" after position [4]: ${strMovieTitle.includes("The", 4)}`); // returns true
This newer method is preferred over the older, slower indexOf() method.
Searching and replacing character(s) in a string
You can search a string for a value and replace it with a new value using the following method.
.replace(searchValue, replaceValue) |
The first parameter is the value to be found. And the second parameter is the value you want to replace it with. |
See the example below.
let strSearch = "It was the best of times. It was the worst of times.";
console.log(`Replaces "times" with "years": ${strSearch.replace("times", "years")}`);
// returns It was the best of years. It was the worst of times.
By default, this method:
- Replaces only the first occurrence of the searched-for string.
- Is case-sensitive.
You can change this by using two regular expression parameters.
- Add the global parameter to replace every occurrences of the searched-for string.
- Add the global parameter to replace all occurences, regardless of letter case.
See the examples below.
console.log(`Replaces "TiMeS" with "years": ${strSearch.replace("/times/gi", "years")}`);
// returns It was the best of years. It was the worst of years.