Learning Goals
At the end of this Lesson you will be able to:
- Declare string, numeric and Boolean variables, and assign values to them with the let and const keywords.
- Apply the correct variable naming standards
- Apply the assignment operator correctly in statements that contain variables and values, and variables only.
- Reveal a variable's type using the typeof operator.
In this Lesson you will meet the following terms:
- Variable
- String variable
- Numeric variable
- Boolean variable
- Assignment statement
Exercise Files
For this Tutorial, in your javascript/exercises folder, create a new sub-folder named 2.
Save the exercise file below to this new javascript/exercises/2 sub-folder.
About variables in JavaScript
Update your index.html exercise file by copying the JavaScript snippet below to inside the <script> tags near the bottom of the web page.
let userName; // Declare variable
userName = "Sheila Murphy"; // Assign value to variable
let userCountry; // Declare variable
userCountry = "Ireland"; // Assign value to variable
You can think of a variable as a container or ‘storage box’ for a value that may be different each time the JavaScript code is run.
Consider the example of a website login page that asks for a user's First Name.
The value entered might be 'Laurent' or 'John' or whatever. But in the JavaScript code that stores the value, the variable will always have the same name of, for example, userFirstName.
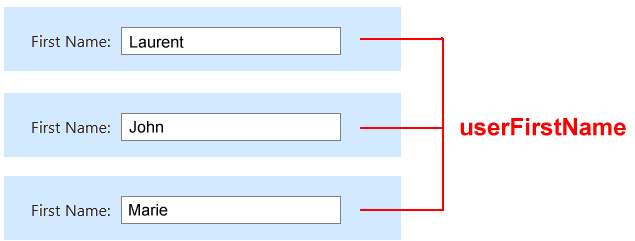
In JavaScript the first thing you do with a variable is declare it. The following code declares the variable named userFirstName.
var userFirstName; // Declares (creates) a new variable
The variable declaration begins with the word var. This so-called keyword allocates memory storage space for new data and tells JavaScript that a new variable name is in use.
The more modern version of JavaScript uses the keywords let or const to declare a variable. For example:
let userFirstName; // Modern JavaScript variable naming
const |
Use this for a variable where the value stored remains constant when the script is run. |
let |
Use this for a variable where the value stored may change during the running of the script, such as in a math calculation or a loop. |
Variable names
Here are a few points to remember about variable names:
- Uniqueness: Don't use the same name for two different variables within the same scope of JavaScript code.
- No spaces: Variable names cannot contain spaces. For example:
const Product Name; // Invalid variable name let Sub Total; // Also invalid variable name
- Mixed character case: You can combine upper and lowercase letters in a variable name to make it easier to read. For example:
const postCode; // camel case variable name let localCurrency; // Also camel case variable name
- Case sensitivity: Variable names are case-sensitive. For example:
const firstName; // This is one variable name let FirstName; // This is a DIFFERENT variable name
When you declare a variable you will often want to assign a value to it. See the example below.
let userName; // Declare variable userName = "Sheila Murphy"; // Assign value to variable let userCountry; // Declare variable userCountry = "Ireland"; // Assign value to variable
You can minimise typing by declaring a variable and assigning a value to it in the same statement on the same line. Here’s two examples.
let userEmail = "Sheila Murphy"; let userPassword = "banana42%";
When you declare a variable with const, you must assign a value to it at the time your declare it. For example:
const userLastName = "Murphy";
If you simply declare a variable with const, you can see an error message in the JavaScript Console. For example:
const userLastName; // Error. New const variable is undefined (contains no assigned value)

About string variables
The variables you have worked with so far have all been of one type: the string variable. This is a variable type that holds letters, numbers, blank spaces or punctuation symbols.
When you enclose the value in single quotes (''), double quotes ("") or backticks (``) JavaScript assumes that you want to create a string variable. For example:
const firstName = "Peter"; let lastName = 'Smith'; let streetAddress = `42 Green Avenue`;
You will learn more about string variables in the Working with strings Tutorial.
About numeric variables
Another type of variable in JavaScript is the numeric variable, which stores a number that you can perform arithmetic operations on
When you omit the quotes around the value, JavaScript makes the variable a numeric variable. For example.
let orderQty = 42; let productPrice = 9.99;
Unlike most programming languages JavaScript does not have different types of numeric variables for different types of numbers. All numbers, whether integers (whole numbers) or floating-point numbers (numbers with decimal places) are all stored within the one type of numeric variable type. Numeric variables may store ether positive or negative numbers.
You will learn more about numeric variables in the Working with numbers Tutorial.
Here are two values that JavaScript will accept as valid numbers.
let Temperature = -6.3456; let errorRate = .2727;
And here are two values that are not valid numbers in JavaScript.
let userIncome = 34,000 // Error. Contains a comma. let product ID = 645w29 // Error. Contains a letter character.
In each case, the JavaScript Console will show the following error message.

When declaring a variable you don’t need to tell JavaScript which type of variable you want to create. JavaScript decides the variable type according to the type of value you assign to it.
Variable
A container that can store a changing value. A variable may contain strings or numbers. Variable names must be unique, are case sensitive and cannot contain spaces.
Boolean variable type
A third type of variable in JavaScript is the Boolean variable. This may have one of only two possible values: true or false.
Developers typically give Boolean variables names such as isCustomer, isLoggedIn, isSelected, and so on.
Automatic variable retyping
JavaScript, as you have learnt, decides which type of variable you want to create by the way that you first assign a value to it. When you enclose the value within quotes, JavaScript makes the variable a string variable; when you omit the quotes, JavaScript makes it a numeric variable.
JavaScript can also change the type of a variable when you assign a value of a different type to that variable. It's called automatic type conversion. Consider the following two statements.
let myvar = "12.34"; myvar = 12.34;
In the first statement JavaScript makes the variable named ‘myvar’ a string variable. In the second statement JavaScript changes the variable type to numeric because a number was assigned to it.
Automatic variable type conversion is a powerful feature of JavaScript, but its very power can result in scripting errors or in scripts that produce unexpected results. You need to be particularly careful with variables types when dealing with HTML forms that accept input from web page visitors. Because forms accept input as strings you need to convert such strings to numbers when you want to include them in arithmetic calculations.
About the assignment (=) operator
Putting a value into a variable is known as assignment, and is achieved through the use of the assignment operator (=). For example:
fruit = "apple";
This places the value of ‘apple’ inside the variable named ‘fruit'. Switching around this assignment statement results in the value of ‘fruit’ being placed inside the variable named ‘apple'.
apple = "fruit";
In JavaScript the equals sign does not mean 'equal to' as it does in arithmetic or algebra. It means as follows:
‘Take whatever is on the right of the equals sign and place it in variable on the left hand side.'
JavaScript treats the left-hand side (LHS) as a variable, but the right-hand side (RHS) may be a value, another variable, or an object property. In the example below the RHS contains a value.
country = "Bermuda";
Consider the next example. Here the RHS contains another variable. What this assignment does is copy whatever value is in the ‘cost’ variable to the ‘price’ variable.
price = cost;
It's perfectly valid to place the same variable on both sides of the assignment operator. The following example puts the current content of the StreetAddress variable inside the StreetAddress variable. Nothing is changed as a result of this statement but no syntax rule is broken either.
StreetAddress = StreetAddress;
Sometimes you may want to change a variable's value by a fixed amount. The following would make no sense if it where algebra.
SalePrice = SalePrice + 20;
But in JavaScript it means ‘jack up the sale price by 20'. That is, insert in the sale price variable whatever is currently in that variable already plus 20.
JavaScript does not accept an assignment statement with a value on both sides. The LHS must always be a variable or an object property. Running the following statement generates an error in the web browser window.
32 = 32;
Assignment statement
A JavaScript statement in which a variable or object property on the left of the equal sign (=) is given the value of whatever is on the right of the equals sign.
Mixing literals and variables
Often you will want to includes literals and variables in the same JavaScript code. Literals are unchanging items of text, numbers or symbols.
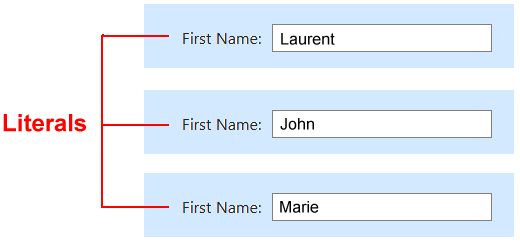
In the past, the only way to join or concatenate literals and variables was to use the + operator. See the examples below.
const userName = 'Marie';
const balance = 10';
// Using string concatenation
const str1 = 'Hi ' + userName + ',' + ' your balance is ' + balance + '.'
console.log("Regular string: ", str1)
Note how using concatenation with the + operator involves adding many plus signs. You must also account for whitespace and escaping certain characters such as " with \".
Modern JavaScript uses so-called template literals. With thid method, there was no need to add any plus signs. You write everything together as a single string. You can directly embed variables with the ${} syntax. See below.
const userName = 'Marie'; const balance = 10'; // Using string concatenation const str1 = 'Hi ' + userName + ',' + ' your balance is ' + balance + '.' console.log("Regular string: ", str1); // Using template literals const str2 = `Hi ${userName}, your balance is ${balance}.`; console.log("Template literal: ", str2);
Another way template literals make it easier to work with strings is when dealing with multi line strings. For regular strings, you have to use a combination of the plus + sign and \n to denote a new line. But template literals don't require any of that.
const regularString = "Hello there! \n" + "Welcome to our website. \n" + "How can we help you today?"; const templateLiteral = `Hello there! Welcome to our website. How can we help you today?` console.log(regularString) console.log(templateLiteral)