Learning Goals
At the end of this Tutorial, you will be able to:
- Use the <script> tag to include JavaScript code in a HTML file and to link to external JavaScript files.
- Ensure that JavaScript code is non-blocking — it does not delay the loading of the HTML content.
- Ensure that JavaScript code does not attempt to access the HTML content until the HTML has been fully loaded.
- Open and use the JavaScript Console in your web browser’s DevTools.
Exercise Files
To work with the exercise files in this and later Tutorials:
- Create a new folder named javascript.
- In this, create a sub-folder named exercises.
For this Tutorial, in your javascript/exercises folder, create a new sub-folder named 1.
Save the two exercise files below to this new javascript/exercises/1 sub-folder.
📄 index.html
📄 script-1.js
JavaScript and the <script> tag
The most efficient ways to add JavaScript to a web page are as follows:
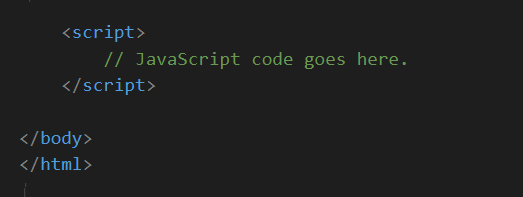
If your JS code is in the HTML file, add it to the bottom before </body> tag. Script loads after web page has been fully loaded.
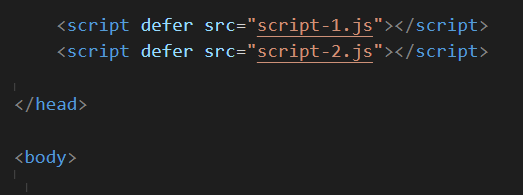
Add external JS file(s) to the head with defer attribute. Files load ‘in the background’ in order placed. Only run after web page is fully loaded.
Both methods are:
- Non-blocking: Loading the JS code does not delay rendering of the HTML. So users experience faster page load time. And you should see fewer audit messages like this on Google's PageSpeed Insights site.
- Access to HTML elements: The JS code will try to access elements in the HTML file only after the file is loaded. So you should not see errors like this in the JavaScript Console.
External JavaScript files
Here are the benefits of using external JavaScript files:
- They separate HTML and JavaScript code.
- They make the HTML and JavaScript easier to read and maintain
- On multi-page websites, cached JavaScript files can speed up page loads.
Do not include <script> tags in external JavaScript files.
Working with web browser DevTools
Your web browser’s Developer Tools (also known as DevTools) includes a JavaScript Console. To display this:
- Windows (all browsers): Click the three dots icon at the top-right of your browser window. From the dropdown menu displayed, click More tools to display a second menu. On that menu, click the last option, Developer tools.
Alternatively, press Ctrl + Shift + I
- Mac (Safari browser): Choose Safari | Preferences, click Advanced, then select Show Develop menu in menu bar. Alternatively, press ⌘ + ⌥ + I.
Changing the DevTools position
To position or ‘dock’ the DevTools window:
- Open the DevTools window.
- Near the top-right of the DevTools window, click the ellipses (three dots) icon.
- Click the docking position you want for the DevTools window.
Below is an example of the DevTools window docked on the right.
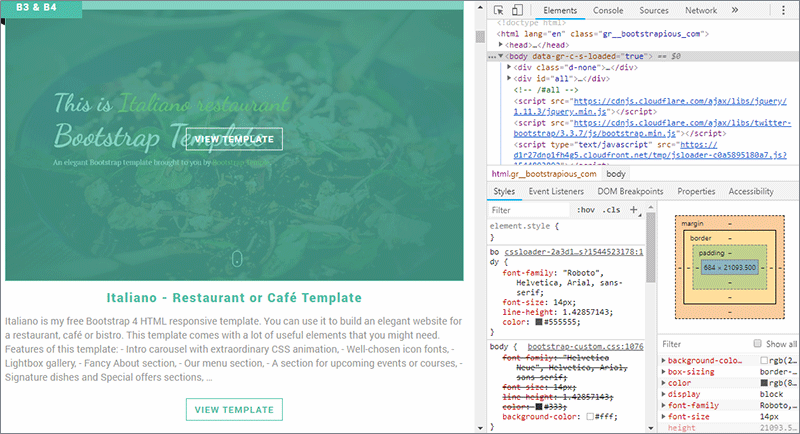
And here is an example of the DevTools window docked along the bottom.
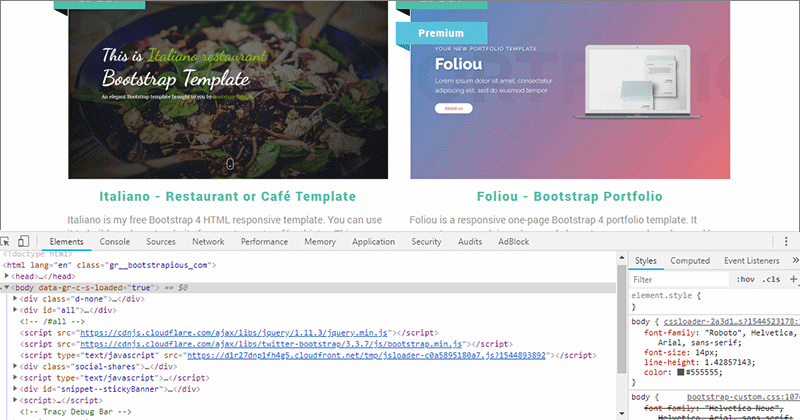
Changing the DevTools font size
To change the font size in the DevTools window, select the window, and then press the Ctrl key followed by the plus (+) or minus (-) key. To reset, press Ctrl and 0.
Working with the JavaScript Console
Of the various tabs shown across the top of DevTools window, click Console to display the JavaScript Console.
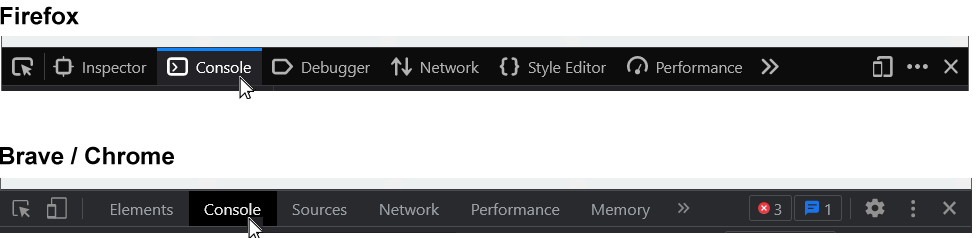
You can use the Console to display or ‘log’ information as part of your JavaScript coding process.
- Any coding errors will appear in the Console, along with the line number and a short explanatory message.
- In your code, you can use console.log() statements to inspect the values of variables and properties of objects.
In summary, the JavaScript Console provides an environment similar to a terminal shell interface to try out JavaScript code in real-time.
JavaScript and AI tools
There are lots of AI tools to help you on your JavaScript learning journey. For example:
With these services, you will obtain better, more focused results if you begin by entering a persona such as the following:
You are a skilled and experienced JavaScript developer and instructor. You have
a special skill in explaining modern ES5/ES6 JavaScript concepts, features and best
practices. Please respond to my questions and requests with basic,
step-by-step directions supported by sample code snippets.
Please display "read" to acknowledge. Thank you.
In VS Code, install the following two extensions:
- GitHub Copilot
- GitHub Copilot Chat
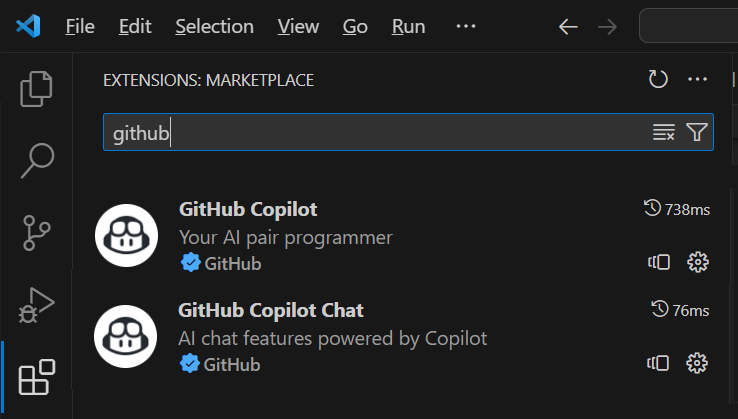
Exercises
Follow these steps:
- Open the index.html web page. Just before the closing </body> tag, enter the following JavaScript code snippet:
- Save and reload the web page. You should see the following message in your browser's JavaScript Console:
- In the index.html file, add the following line just before the closing </head> tag.
- Open the script-1.js file and add the following new line:
- Save the JavaScript file and reload the web page. You should see the following message in the JavaScript Console: